Journeying Through Big-O Notation
-
Jared Swanson
- January 10, 2023
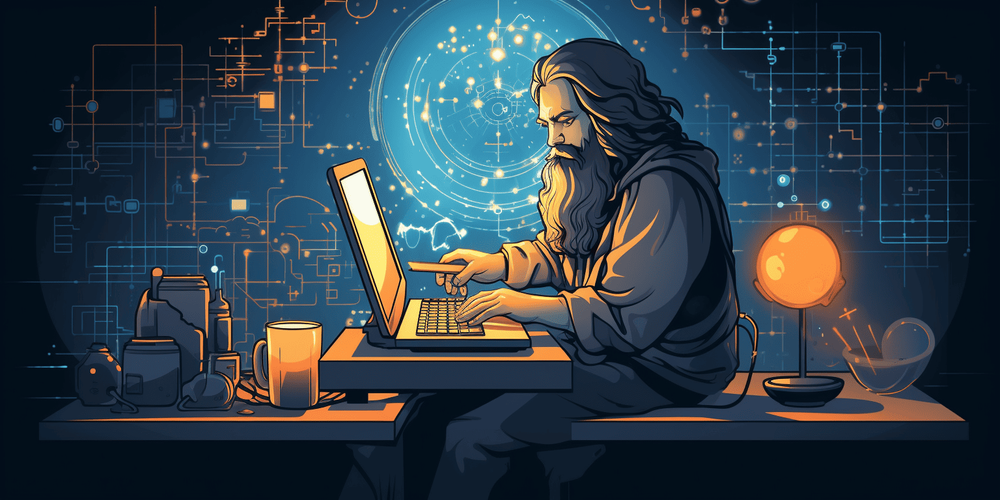
In the realm of computer science and programming, Big-O Notation is an essential concept. It allows you to assess the efficiency of your code and predict how it will perform as the input data grows. With this understanding, you can make informed decisions about optimizing your algorithms.
O(1) - The Magic Portal
The notation O(1) denotes a constant time complexity, meaning the algorithm runs in a fixed amount of time regardless of the input size. An example in Ruby is the .first
method on an array, which retrieves the first element regardless of the array’s length.
def magic_portal(arr)
arr.first
end
magic_portal([1,2,3,4,5])
This code represents a magic portal that instantly teleports you to the first element of the array, no matter how long the array is.
O(n) - The Desert Linear Search
O(n) represents linear time complexity, where the running time of an algorithm grows linearly with the size of the input. It describes a one-to-one relationship between the input size and the time taken.
def find_gem_in_desert(desert)
desert.each do |place|
return place if place == "gem"
end
end
find_gem_in_desert(['sand', 'sand', 'gem', 'cactus'])
Here, you’re searching for a hidden gem in a desert. If the desert has n places, in the worst-case scenario, you might have to visit all of them to find the gem. This illustrates the linear relationship between input size and runtime.
O(n^2) - The Dance of Double Loops
O(n^2) denotes quadratic time complexity, which means that the runtime of an algorithm is proportional to the square of the input size. It usually occurs with nested loops.
def dance_pairings(dancers)
pairs = []
dancers.each do |dancer1|
dancers.each do |dancer2|
pairs << [dancer1, dancer2] unless dancer1 == dancer2
end
end
pairs
end
dance_pairings(['Alice', 'Bob', 'Charlie'])
Imagine a magical dance where every dancer must dance with every other dancer. If there are n dancers, there will be n*(n-1) dance pairings, which grows quadratically as the number of dancers increases.
O(2^n) - The Multiplying Magical Forest
O(2^n) is an exponential time complexity that grows exponentially as the input size increases. Such algorithms become slower much more quickly as the input size grows.
def tree_growth(n)
return 1 if n == 0
2 * tree_growth(n-1)
end
tree_growth(3)
In this example, every tree in a magical forest duplicates itself. Touching 3 trees results in 8 trees due to their exponential growth.
O(n+m) - The Quest Across Two Kingdoms
O(n+m) indicates linear time complexity across two separate inputs. It describes algorithms where two different inputs need to be processed individually.
def quest_across_kingdoms(kingdom1, kingdom2)
kingdom1.each { |place| visit(place) }
kingdom2.each { |place| visit(place) }
end
In this quest, you visit every place in two kingdoms, kingdom1 and kingdom2. The time taken is proportional to the total number of places in both kingdoms.
O(n*m) - Trading Between Kingdoms
O(n*m) signifies that the runtime is proportional to the product of the sizes of two different inputs.
def trade_deals(merchants_kingdom1, merchants_kingdom2)
deals = []
merchants_kingdom1.each do |merchant1|
merchants_kingdom2.each do |merchant2|
deals << [merchant1, merchant2]
end
end
deals
end
Here, every merchant in one kingdom trades with every merchant in another kingdom. If there are n merchants in the first kingdom and m in the second, there will be n*m trade deals.
To summarize, Big-O Notation is an invaluable tool for evaluating algorithm performance. By understanding how different complexities scale with input size, you can optimize your code more effectively. This knowledge, illustrated through our themed examples, will empower you to tackle coding challenges with more insight and creativity.