High School Gossip and the Law of Demeter
-
Jared Swanson
- June 7, 2022
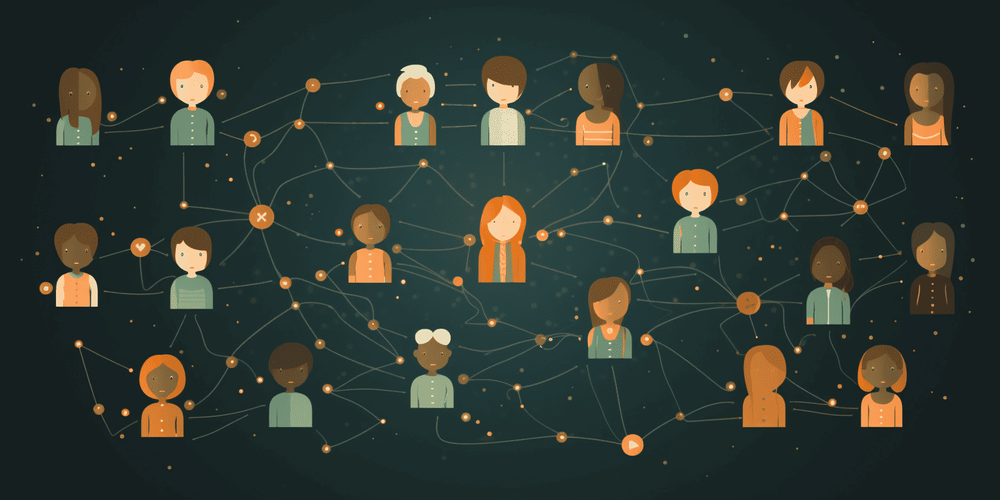
High school halls are abuzz with whispers. Stories evolve, facts get distorted, and by the time a piece of gossip has reached its tenth listener, it’s almost unrecognizable. But what if there was a way to ensure that information remains accurate? This is where the Law of Demeter (LoD) or the “principle of least knowledge” comes into play. In programming, just like in high school gossip, the LoD helps in reducing misunderstandings by ensuring direct communication.
What is the Law of Demeter?
At its core, the Law of Demeter encourages objects to only communicate with their immediate “friends” or closely related objects. An object should not inquire about the details of other objects it doesn’t directly know.
Why Should We Care About the LoD in Ruby?
- Reduced Dependencies: By communicating directly with only closely related objects, you reduce the dependencies, making the code more maintainable.
- Enhanced Encapsulation: Objects hide their internal details, leading to better encapsulation.
- Clearer Code: Code becomes more readable, as you’re not traversing through long chains of method calls.
Let’s Explore Gossip at Ruby High School
Direct Communication vs. The Gossip Chain
Let’s model a situation where a student tells a gossip directly to their best friend versus passing it through multiple intermediaries.
class Student
attr_accessor :name, :best_friend, :acquaintance
def initialize(name)
@name = name
end
def tell_gossip_directly(message)
best_friend.hear(message)
end
def pass_gossip_through_chain(message)
best_friend.acquaintance.hear(message) if best_friend&.acquaintance
end
def hear(message)
puts "#{name} heard: '#{message}'"
end
end
alice = Student.new('Alice')
bob = Student.new('Bob')
charlie = Student.new('Charlie')
alice.best_friend = bob
bob.acquaintance = charlie
# Alice tells Bob directly
alice.tell_gossip_directly("Did you hear about the surprise test?")
# Output: Bob heard: 'Did you hear about the surprise test?'
# Alice passes the gossip through Bob to Charlie
alice.pass_gossip_through_chain("I think there's a surprise test!")
# Output: Charlie heard: 'I think there's a surprise test!'
By employing a chain, Alice indirectly communicates with Charlie through Bob, showing the potential pitfalls of long communication chains. Messages can get altered or misunderstood as they travel through intermediaries.
Applying the Law of Demeter
Now, let’s refactor the above code to adhere strictly to the LoD. This will simplify and improve the communication chain.
class Student
attr_accessor :name, :best_friend
def initialize(name)
@name = name
end
def tell_gossip(message, target_friend = best_friend)
target_friend.hear(message)
end
def hear(message)
puts "#{name} heard: '#{message}'"
end
end
alice = Student.new('Alice')
bob = Student.new('Bob')
charlie = Student.new('Charlie')
alice.best_friend = bob
bob.best_friend = charlie
# Alice tells Bob directly
alice.tell_gossip("Did you hear about the surprise test?")
# Output: Bob heard: 'Did you hear about the surprise test?'
# Alice passes the gossip through Bob to Charlie
bob.tell_gossip("Alice said there's a surprise test!")
# Output: Charlie heard: 'Alice said there's a surprise test!'
In this improved version, Alice tells Bob, and Bob then tells Charlie. While it’s a small and subtle change, this emphasizes the principle of each student speaking directly to their best friend, aligning more closely with the Law of Demeter.
In Conclusion
Whether it’s the hallways of high school or the lines of a Ruby program, direct communication reduces the chance of misunderstandings. By adhering to the Law of Demeter, we can ensure that our objects have clear, concise interactions, resulting in more reliable and maintainable code. Keep your conversations direct and your code will thank you! 📣🎓