What is Ruby's Modulo Operator?
-
Jared Swanson
- February 15, 2023
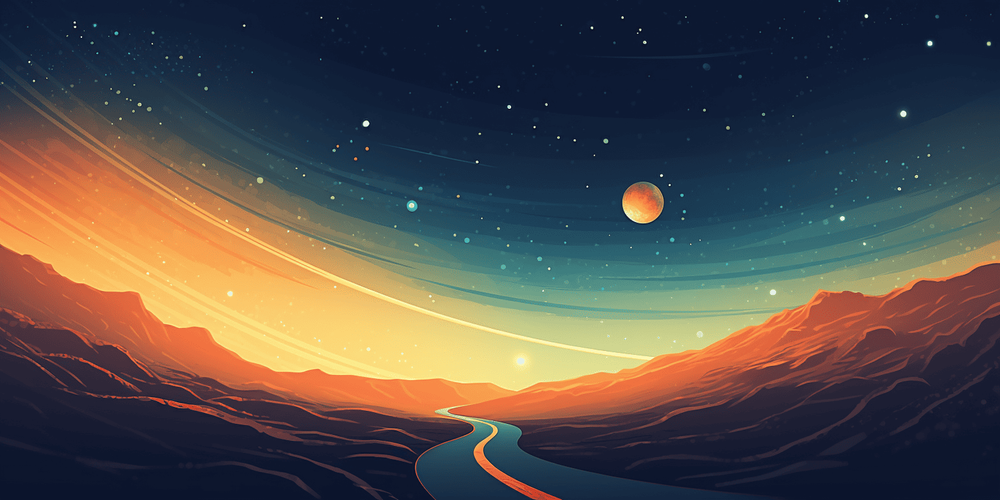
🚀 🌌 Attention all space travelers! When you’re charting a course through the stars, you’re bound to encounter the modulo operator, represented as %
, a versatile mathematical tool that returns the remainder of a division operation. With it, you can easily find out if a number is divisible by another or perform actions at regular intervals.
Blast Off with Modulo Basics
The modulo operator’s primary function is to return the remainder of a division. Among other things, it’s a handy way to check if a number is even or odd. In Ruby, you can easily find this out with the built-in even?/odd?
methods.
100.even? # true
99.even? # false
But what if you need to check divisibility by numbers other than 2? Fear not, brave explorer! The modulo operator is here to help.
(1..30).each do |day|
puts "Stargazing tonight!" if day % 5 == 0
end
Output:
Stargazing tonight!
Stargazing tonight!
Stargazing tonight!
Stargazing tonight!
Stargazing tonight!
Stargazing tonight!
In this example, we use the modulo operator to schedule stargazing nights every 5 days.
Spaceworthy Uses of Modulo Operator
Divisibility
When charting a course through the cosmos, it’s important to know if a number is divisible by another. If the remainder of the division is zero, the number is considered divisible.
Alien Name Translator
alien_names = ["Zog", "Blip", "Gleep", "Zap"]
alien_names.each_with_index do |name, i|
puts "Greetings, #{name}!" if i % 2 == 0
end
Output:
Greetings, Zog!
Greetings, Gleep!
In this example, we use the modulo operator to greet every other alien in our list.
Intergalactic Disco
(1..12).each do |hour|
puts "Disco time!" if hour % 3 == 0
end
Output:
Disco time!
Disco time!
Disco time!
Disco time!
In this example, we use the modulo operator to have a disco every 3 hours.
Performing Actions at Regular Intervals
The modulo operator is a space navigator’s best friend when it comes to performing actions every nth time. Use it to select every nth item from a list:
(3..15).select { |n| n % 5 == 0 }
# [5, 10, 15]
In this example, we use the modulo operator to select planets that we will visit every 5 days.
Or make use of the step
method:
(0..60).step(12).to_a
# [0, 12, 24, 36, 48, 60]
In this example, we use the step
method to step through every 12th night.
Time Conversion
Navigating the cosmos requires precise timekeeping. The modulo operator comes in handy for converting units of time, like turning minutes into hours and remaining minutes:
Interstellar Travel Duration
days, hours = 1000.divmod(24)
# [41, 16]
In this example, we use the divmod
method to find out how many days and hours it will take to reach a distant star.
Warp Speed Calculation
warp_factor, remainder = 299792458.divmod(1000000)
# [299, 792458]
In this example, we use the divmod
method to calculate the warp factor needed to travel at the speed of light and the remainder of speed.
Back to Earth
In conclusion, the Ruby modulo operator is an invaluable tool in the hands of any space explorer. Whether you’re checking divisibility, scheduling regular activities, or converting units of time, this operator is your trusty sidekick in the vast expanse of space. It can also join forces with other mathematical concepts, like number systems and bitmasking, to tackle a universe of problems.